How I became a full-stack developer
I didn’t know how to learn to code
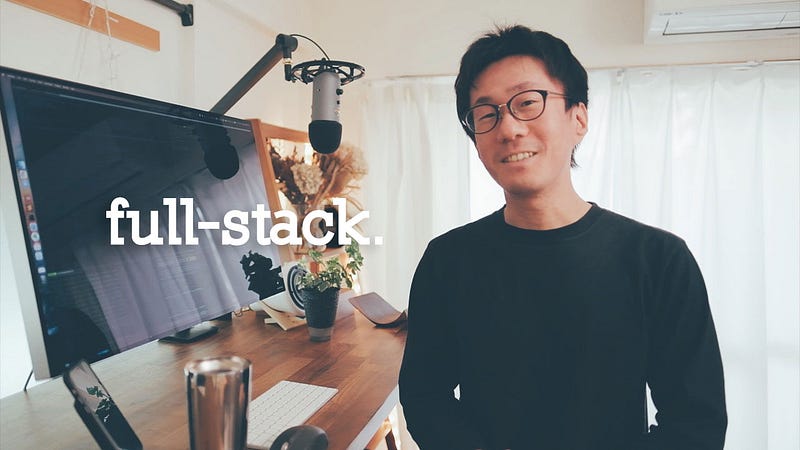
Learning to program is hard. It takes a long time. People seem to be trying hard to find a shortcut to learn it as fast as possible in a smart way. That makes sense because you don’t want to waste your time. But, that would be a pitfall. You don’t have to be so “clever” to master coding efficiently.
Let me tell you what that means.
Hi, I’m Takuya, a full-stack developer from Japan. I built a whole app alone, and I can write in almost any language I need. For example, I’ve written code in JavaScript, Java, Kotlin, Objective-C, Swift, and C++ for building my React Native app. I’ve also written Python and Lua to create a video art using DeepLearning. There is no strict definition of “full-stack” but, in my opinion, being a full-stack developer doesn’t mean you know everything.
Being a full-stack developer means that you can quickly adopt new technologies as much as you need as if you know everything.
Watch video: https://youtu.be/EC-FPKmFxr4
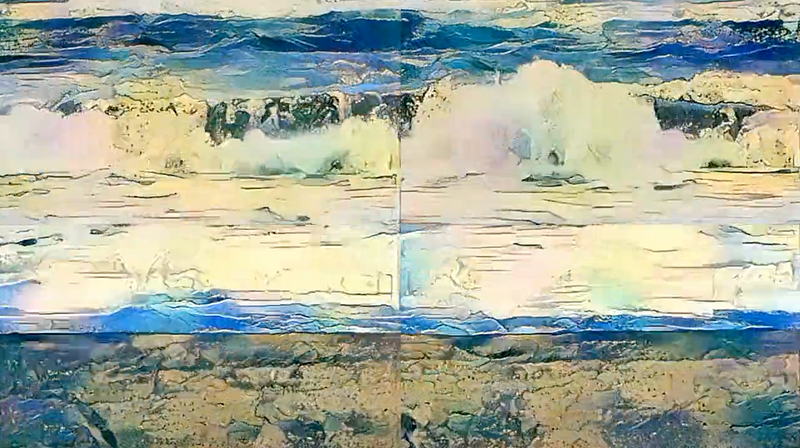
Understand the programming paradigms
Why is it possible? Because there are no significant differences between programming languages. The paradigms of coding are basically the same, such as Object-Oriented Programming, data structure, multithreading, etc.
For example, function signatures are similar regardless of languages.
Say, you got to find the index of the element in a certain array. In JavaScript, the signature of indexOf
method looks like so:
array.indexOf(searchElement[, fromIndex])
The first argument is the element to locate in the array. And the second argument is the index to start the search at. You can call it like so:
['a', 'b', 'c'].indexOf('b') // => 1
['a', 'b', 'c'].indexOf('z') // => -1
It returns the index if found, otherwise, it returns -1
. In Python, the signature looks like this:
list.index(element, start, end)
And you can call it like this:
['a', 'b', 'c'].index('b') # => 1
['a', 'b', 'c'].index('z') # => ValueError: 'z' is not in list
Very similar, right? In PHP, it looks like this:
array_search ( mixed $needle , array $haystack , bool $strict = false ) : int|string|false
array_search('b', ['a', 'b', 'c']); // => 1
array_search('z', ['a', 'b', 'c']); // => false
If you already know indexOf
method of JavaScript, it is not hard to understand how to call array_search
in PHP or list.index
method in Python. Right? So, as you understand a particular language deeply, learning another language can be easy by reusing your knowledge and experience.
This technique can be applied to frameworks as well.
Once you’ve learned React, you can reuse the knowledge for learning another similar framework like Yew of Rust. You can write a component something like this in Yew:
use wasm_bindgen::prelude::*;
use yew::prelude::*;
struct Model {
link: ComponentLink<Self>,
value: i64,
}
enum Msg { AddOne }
impl Component for Model {
...
fn view(&self) -> Html {
html! {
<div>
<button onclick=self.link.callback(|_| Msg::AddOne)>{ "+1" }</button>
<p>{ self.value }</p>
</div>
}
}
}
So, if you are already used to writing React, you should be able to understand Yew framework quickly. You will notice that there are a lot of common designs and paradigms between languages like that. That's because the developer communities are influencing each other.
My favorite one is that the idea of JavaScript's async/await
has come from C#. It's interesting, isn't it? So, most ideas are the mixture of the existing ideas.
Stick with a particular stack
The point is that you should be deeply into a particular language and framework. In fact, how many languages and frameworks you can use don't matter. But how deeply you understand the language and framework is important. Because you should have tackled to solve difficult problems with them. Those experiences are the true gem that can be reused for other skills.
A lot of people are obsessed to talk about the "best" programming language and the "best" library and framework, etc. That doesn't make sense. Because they actually never exist. Instead, there are the optimal language and library for solving your problem. So, don't blindly choose what they told you.
Choose technologies based on the problem you are trying to solve and stick with them. So, try every technology that looks promising for you and decide which to use by yourself.
Make a lot of mistakes
So, there are a lot of common principles in programming.
And once you chose a dev stack, stick with it so that you can cultivate your own reusable knowledge-base. But how to get that knowledge efficiently?
That is to make as many mistakes as possible. I made countless mistakes while learning to code.
People usually try to avoid making mistakes by reading books and articles on the internet. Of course, knowing the best practices is useful.
Asking people questions is helpful. Yeah, I agree. Those are quick ways to find the answers. But, you will never understand ‘how’ they found the answers. You will never know ‘why’ they do in that way. You are just borrowing their time and effort by asking questions. On the other hand, making a mistake is the opportunity to understand them. Besides, making mistakes can be etched in your memory longer than just remembering the answers. That is something like muscle training.
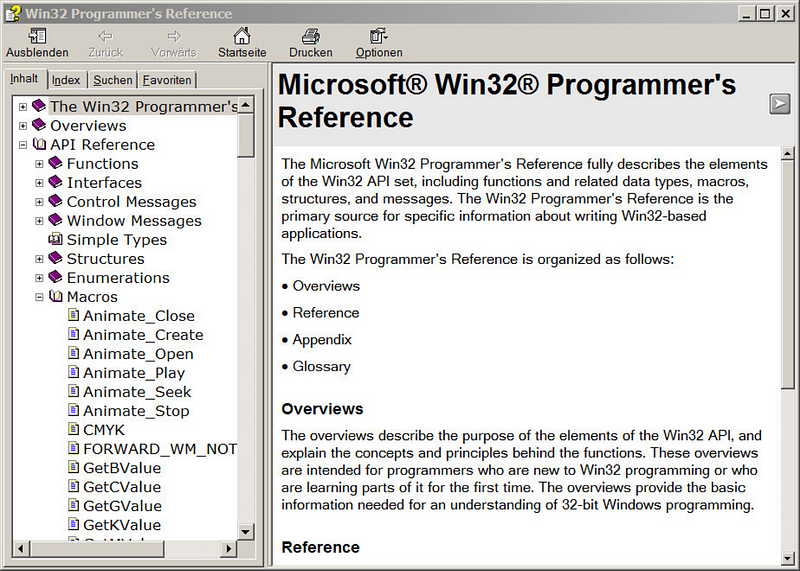
When I started programming at age 13, I didn’t have much money to buy so many books. My home was not connected to the internet yet. So, the Japanese version of the Win32API help file was my bible. I’ve read it and try the Windows APIs again and again without knowing how to call them. Some APIs were not translated and I couldn’t understand the English descriptions, so I tried to specify possible parameters as many as I can come up with until it works. My app and my computer crashed many many times. I was so happy when I finally got it to work. And I’ve learned the API signature patterns little by little. That helped me understand new APIs, and also helped me design interfaces of my functions and methods. That’s how I learned basic design patterns.
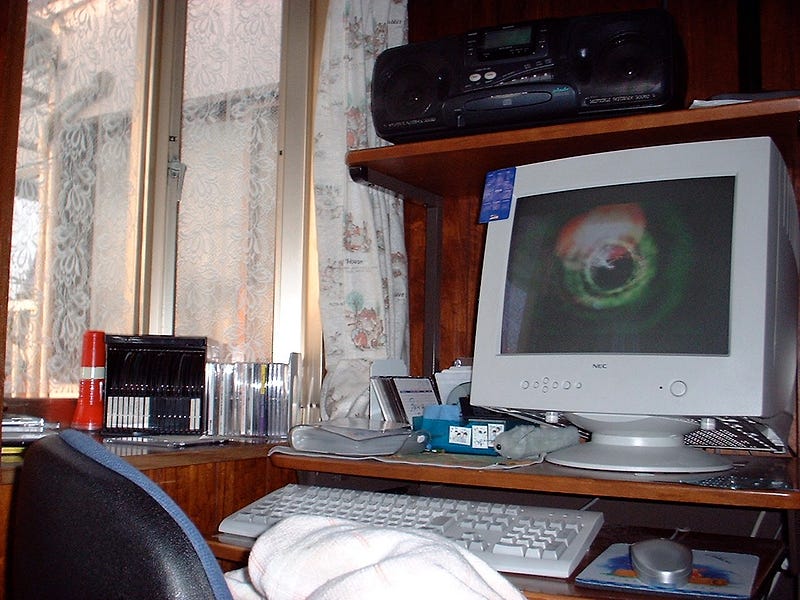
Making a mistake is definitely not embarrassing unlike studying at school.
That’s the necessary step to learn to code. I mean, you need to make mistakes. Don’t be afraid. That’s why I said you don’t have to be so “clever” to master coding efficiently. Cleverly avoiding mistakes is ultimately an inefficient way to learn. You broke your computer by mistake? That’s great. I did it many times, too. You wiped out data from the database by mistake? That’s awesome. I did it many times, too. So, you are doing great. Keep it up!
I don’t mean that you shouldn’t read books or search for answers on Stackoverflow. I mean that you should think with your head and use your hands.
It’s painful but will pay off
Ah, yes, it’s hard. It’s even painful sometimes. It might not be so muchfun when you scratch your head and mumble like, why doesn’t it work, why doesn’t it work..
But, trust me, your effort will pay off.
I was struggling to find good design patterns of Object-Oriented programming. My code was messed up with weird classes. But one day, I suddenly realized how to design classes well. This knowledge doesn’t depend on any OOP languages. And that will make you a full-stack developer.
So, that’s how I learned to code. If you were struggling to learn programming, I hope this video is helpful!
Follow me online
- Inkdrop Website: https://www.inkdrop.app/
- Twitter: https://twitter.com/inkdrop_app
- Instagram: https://www.instagram.com/craftzdog/
- Discord community: https://discord.gg/S7hDmvh
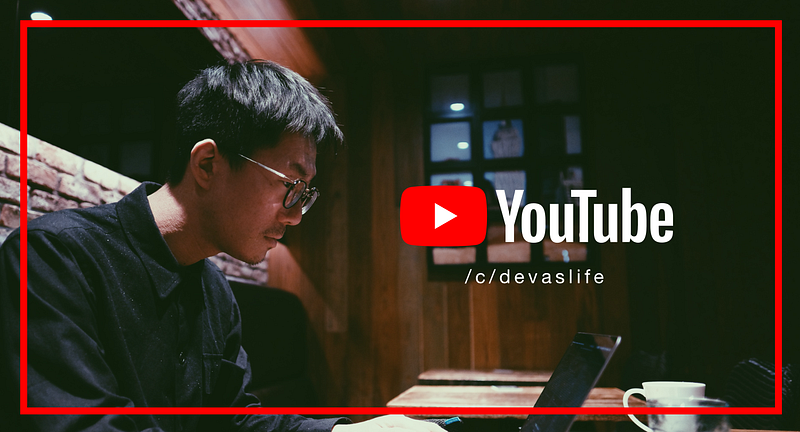
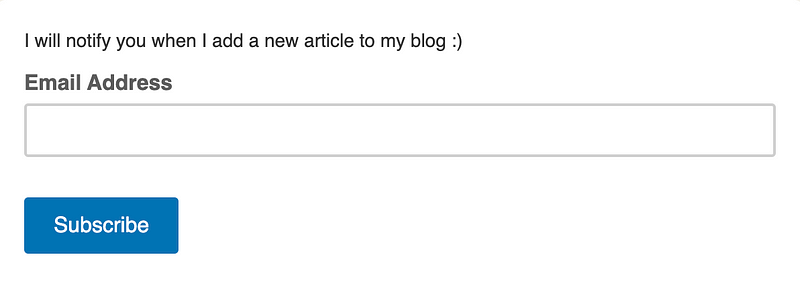