What I did for upgrading React Native to 0.79.1 from 0.74.2
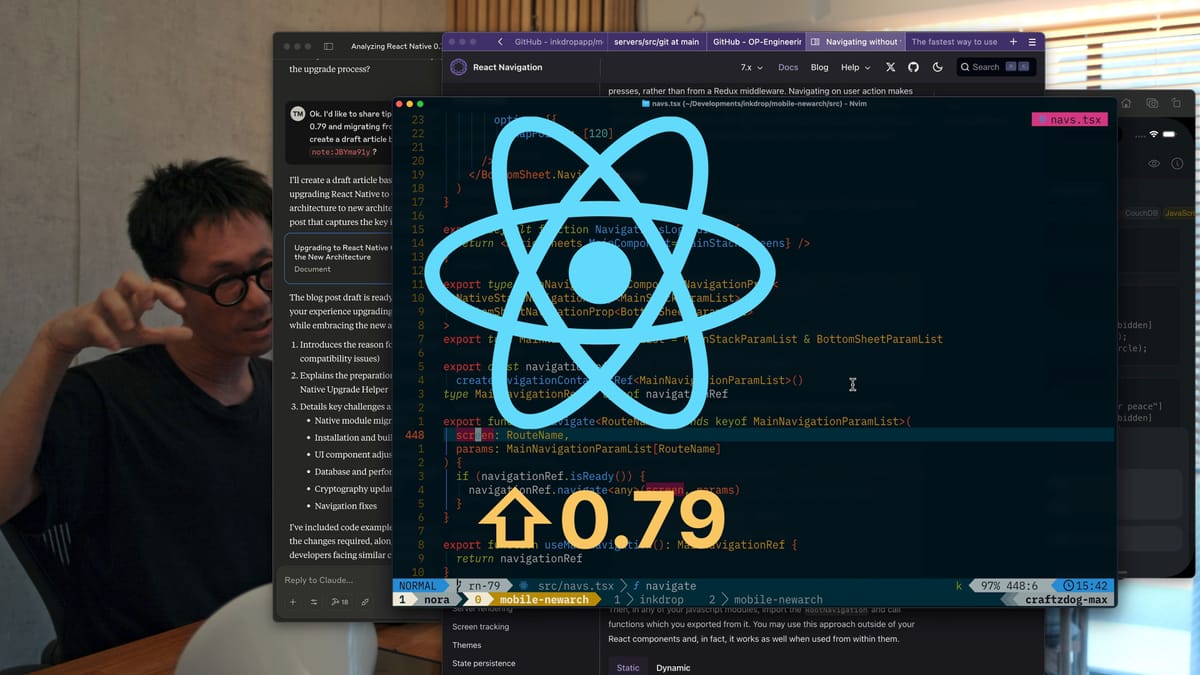
Hi, it's Takuya here, the developer of Inkdrop – a Markdown note-taking app that supports syncing and offline-first capabilities, built with React Native.
Bumping up the React Native versions has always been hard, and this time wasn't an exception, especially because it involved migrating from the old to the new architecture.
So, I'd like to share what I did for this upgrade.
VLOG:
The old RN is no longer able to build with Xcode >= 16.3
As reported here, Xcode 16.3 removed the base template for std::char_traits
.
This change prevents to build iOS app.
So, I could't build my app with React Native 0.74 since it is out of the support window.
So, I had no choice but to upgrade React Native.
Use the upgrade helper
My first step was to visit the React Native Upgrade Helper, which provides diffs between your current version and the target version.
The diffs in my case looked simple. I manually applied them to my project.
After that, I ran into this error when building the app:
[!] CocoaPods could not find compatible versions for pod "RCT-Folly"
I deleted the Podfile.lock
file and ran pod install
again. Then, it solved.
Native module migration
My app has a custom native module for supporting PDF export from an embedded webview.
I shared how to get an instance of the native view components in a custom module here:
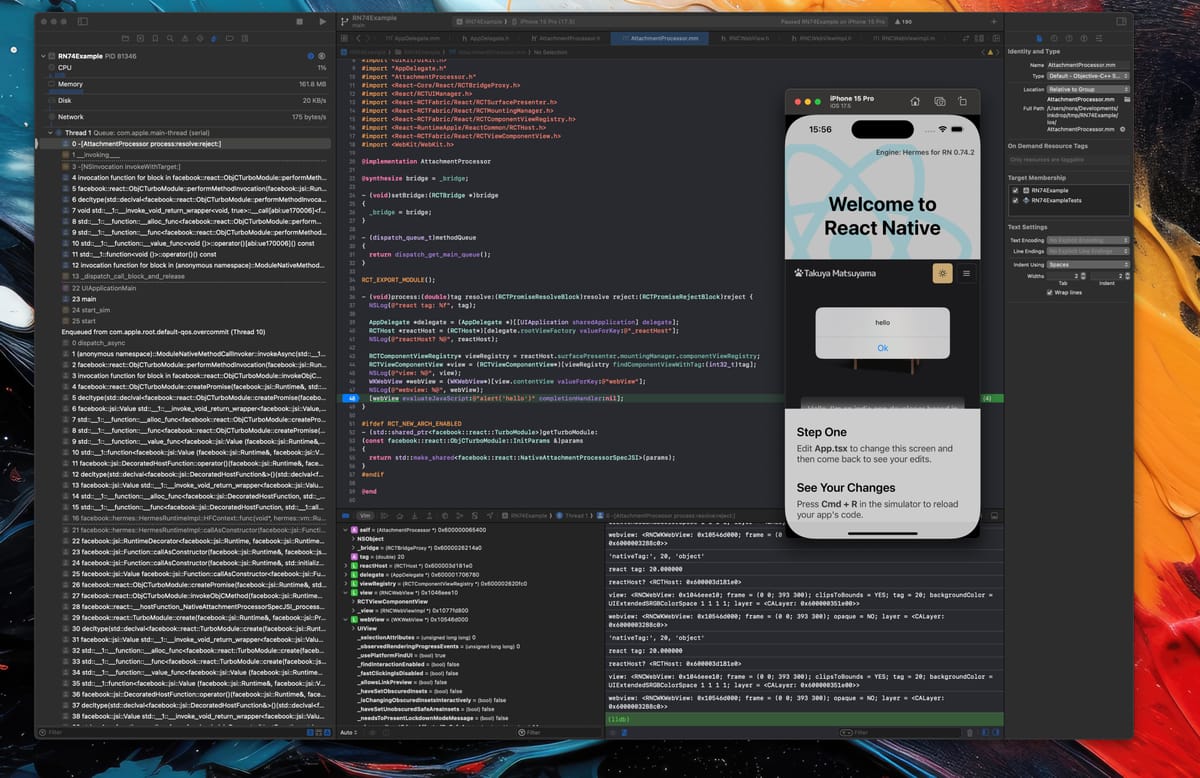
Since then, there have been some breaking changes in internal modules, so I updated the code accordingly.
On Android, reactContext.fabricUIManager
is now deprecated.
Here is the new approach:
// Old approach that no longer worked
val view = reactContext.fabricUIManager?.resolveView(webViewReactTag)
// New approach with UIManagerHelper
val uiManager = UIManagerHelper.getUIManager(reactContext, UIManagerType.DEFAULT)
val view = uiManager?.resolveView(webViewReactTag)
On iOS, the way to get the RCTHost
object has been changed like so:
// Old
AppDelegate *delegate = (AppDelegate *)[[UIApplication sharedApplication] delegate];
RCTHost *reactHost = (RCTHost*)[delegate.rootViewFactory valueForKey:@"_reactHost"];
// New
id<InkdropAppDelegate> delegate = (id<InkdropAppDelegate>)[[UIApplication sharedApplication] delegate];
RCTReactNativeFactory* factory = [delegate reactNativeFactory];
RCTHost *reactHost = factory.rootViewFactory.reactHost;
SafeAreaView
is broken
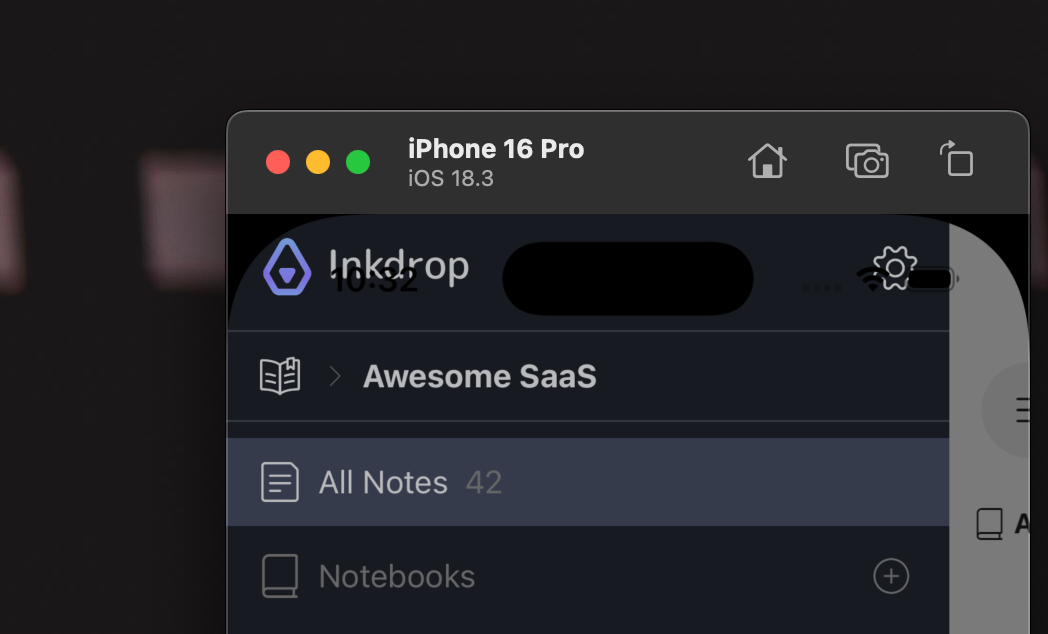
In the sidebar, SafeAreaView
wasn't working correctly as seen in the screenshot above.
I had to add SafeAreaProvider
in the drawerContent
prop like so:
<Drawer.Navigator
initialRouteName="Main"
screenOptions={{
// ...
}}
drawerContent={props => (
<SafeAreaProvider>
<Sidebar {...props} />
</SafeAreaProvider>
)}
>
Bumping up op-sqlite
The app stores data using PouchDB and SQLite as its backing store.
For a SQLite adapter, I use op-sqlite.
There was a breaking change when upgrading it from v6 to v12.
To get it to work as before, you have to change the method as the following:
// Old
const result = await db.executeAsync(query, params);
// New
const result = await db.execute(query, params);
Fixing a bug in react-native-quick-crypto
I made a small contribution to fix a bug in the crypto library:
Updating the pouchdb adapter
I've published a PouchDB adapter for SQLite and shared it in this article:
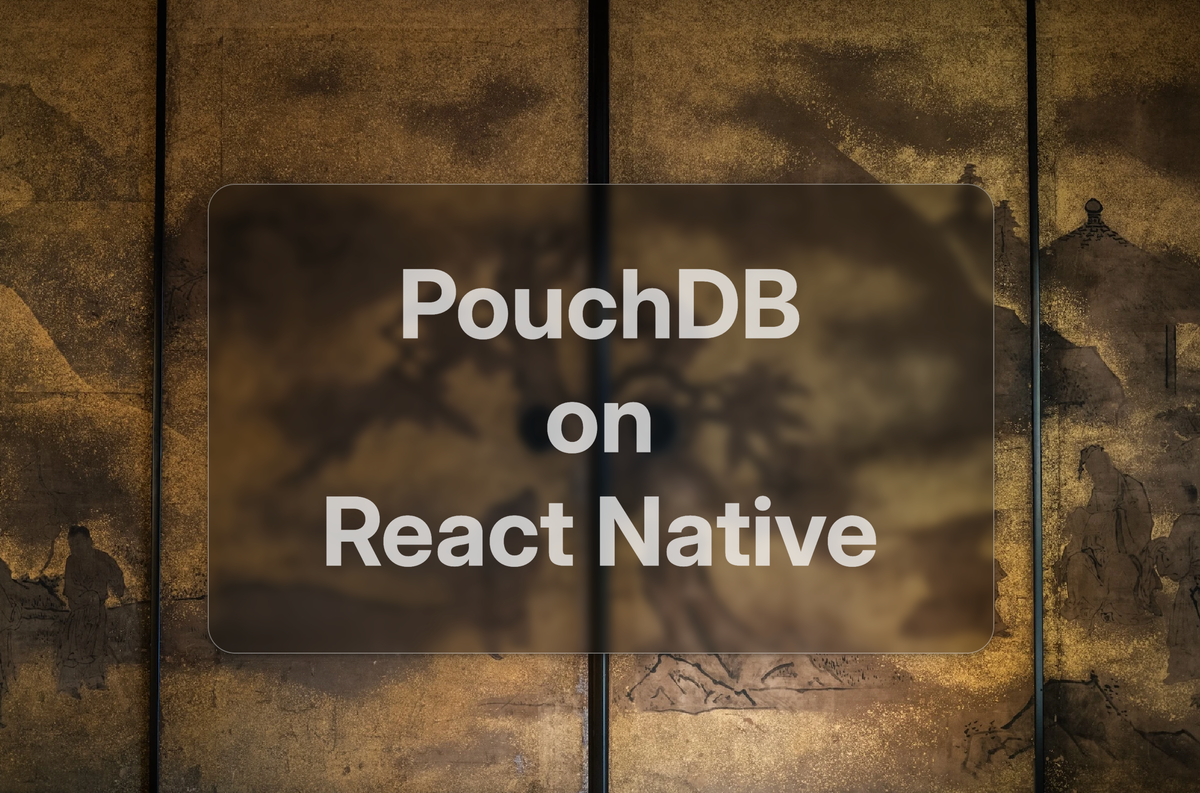
This adapter has been broken on the recent RN versions, so I updated it.
This PR helped me do so (Thanks Gufeng Shen):
I've tested it on my app and it worked fine, so I publishded it:
React Navigation issues
In RN 0.79, some navigations didn't work for some reason.
Maybe this was due to nested navigators.
I found that using createNavigationContainerRef
solved it.
It lets you get the root navigation regardless of the context.
You can use it like so:
// Create a reference
const navigationRef = createNavigationContainerRef();
export default function App() {
return (
<NavigationContainer ref={navigationRef}>{/* ... */}</NavigationContainer>
);
}
export function navigate(name, params) {
if (navigationRef.isReady()) {
navigationRef.navigate(name, params);
}
}
For more information, check out the docs: https://reactnavigation.org/docs/navigating-without-navigation-prop/
iOS App Connect Warning
After updating RN, App Connect started sending this warning when submitting the app:
ITMS-90078: Missing potentially required entitlement - Your app, or a library that's included in your app, uses Apple Push Notification service (APNs) registration APIs...
I greped the source and found that the React Native core uses it:
node_modules/react-native/Libraries/PushNotificationIOS/RCTPushNotificationManager.h:+ (void)didRegisterForRemoteNotificationsWithDeviceToken:(NSData *)deviceToken;
node_modules/react-native/Libraries/PushNotificationIOS/RCTPushNotificationManager.h: * notification.
node_modules/react-native/Libraries/PushNotificationIOS/RCTPushNotificationManager.h: * To process notifications received while the app is in the foreground, call this method from
node_modules/react-native/Libraries/PushNotificationIOS/RCTPushNotificationManager.h: * the push notification should be shown; to match prior behavior which does not show foreground notifications, use
node_modules/react-native/Libraries/PushNotificationIOS/RCTPushNotificationManager.h: * If you need to determine if the notification is remote, check that notification.request.trigger
node_modules/react-native/Libraries/PushNotificationIOS/RCTPushNotificationManager.h:+ (void)didReceiveNotification:(UNNotification *)notification;
node_modules/react-native/Libraries/PushNotificationIOS/RCTPushNotificationManager.h:+ (void)didReceiveRemoteNotification:(NSDictionary *)notification
node_modules/react-native/Libraries/PushNotificationIOS/RCTPushNotificationManager.h:+ (void)setInitialNotification:(UNNotification *)notification;
However, my app doesn't use APNs and PushNotificationIOS
looks already deprecated. Maybe I can ignore the warning:
If there are any ways to avoid this warning, please let me know 🙏
That's it! Most issues were app-specific, but I hope it's helpful.
The new architecture is much more stable than before thanks to the community's effort. Also, it feels like very performant like the faster launch speed.
I will keep focusing on the app quality for providing a great note-taking experience!
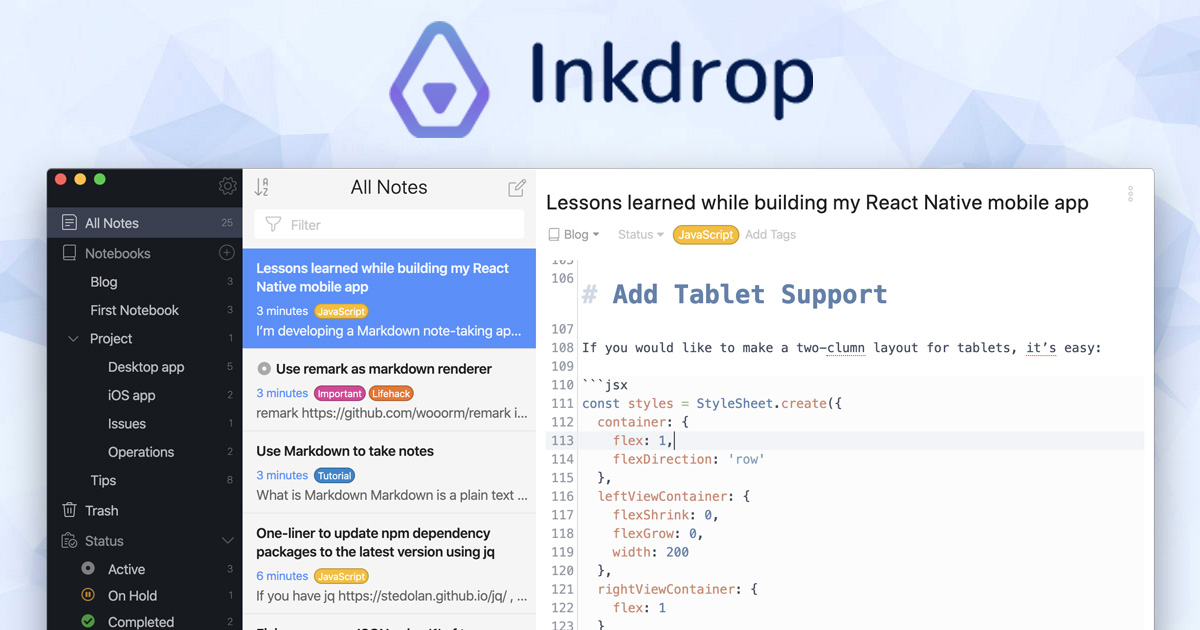